最近在做APP,项目中要用支付,就写了下面的微信支付!还有支付宝支付,看下面的文章!
首先呢,先去开发平台上看看介绍,知道你不想看,但有些东西你是需要滴,
比如说
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| import lombok.Data; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.stereotype.Component;
/** * 微信支付配置文件 * * @author 知吾朱 * @since 2019/7/11 */ @Data @Component @ConfigurationProperties(prefix = "weixin") public class WxProperties {
private String appId;
private String mchId;
private String key;
private String notifyUrl;
private String projectDomain; }
|
这些东西都是你需要的。
1 2 3 4 5 6
| weixin: appId: 不告诉你,自己去拿 key: 还是不告诉你,自己去拿 mchId: 自己去拿,听见没 notifyUrl: /pay/weixin/notify 回调接口 project-domain: http://localhost:12900 你的api域名
|
然后呢,然后就开始敲代码了。
话不多说,上代码。
有一堆堆文件,你可以在微信文档里边下载到。
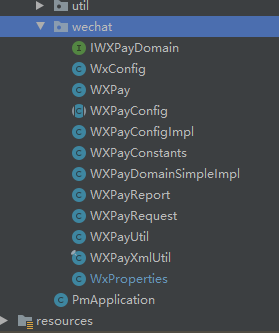
WxProperties就是上边的文件,其他的都可以下载到。
方法送上
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| @Override public Map<String, String> unifiedOrder(String body, String orderNumber, String totalFee, String spBillCreateIp) throws Exception { //构造请求参数数据 Map<String, String> data = new HashMap<>(); //货币类型 data.put("fee_type", "CNY"); //回调地址 data.put("notify_url", wxProperties.getNotifyUrl()); //交易类型 data.put("trade_type", "APP"); //商品描述 data.put("body", body); //订单编号 data.put("out_trade_no", orderNumber); //支付金额 data.put("total_fee", String.valueOf(new BigDecimal(totalFee).multiply(new BigDecimal(100)).setScale(2, BigDecimal.ROUND_HALF_UP).intValue())); //支付IP data.put("spbill_create_ip", spBillCreateIp); return wxPay.unifiedOrder(data); }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| try { Map<String, String> resultMap = chargingOrderService.unifiedOrder( "XXXX-支付测试", "订单编号(唯一的,自己生成)", "钱哦 ,可以填0.01,测试是要花钱的", "你的ip地址,网上有的是方法"); String returnCode = resultMap.get("return_code"); if (Constant.SUCCESS.equals(returnCode)) { String resultCode = resultMap.get("result_code"); if (Constant.SUCCESS.equals(resultCode)) { String prepayId = resultMap.get("prepay_id"); String nonceStr = resultMap.get("nonce_str"); // 构造小程序端调用支付所需参数 Map<String, String> payParam = chargingOrderService.chooseWxPayMap(prepayId, nonceStr); return ApiResponse.success().and("payParam", payParam); } } return ApiResponse.fail().msg(resultMap.get("err_code_des")); } catch (Exception e) { e.printStackTrace(); return ApiResponse.fail().msg(ResponseMsgConstant.NULL); }
|
别急,还有呢,你要回调哦,记住回调要用外网能访问到的,微信调你的。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74
| /** * 返回成功xml */ private static final String RES_SUCCESS_XML = "<xml><return_code><![CDATA[SUCCESS]]></return_code><return_msg><![CDATA[OK]]></return_msg></xml>";
/** * 返回失败xml */ private static final String RES_FAIL_XML = "<xml><return_code><![CDATA[FAIL]]></return_code><return_msg><![CDATA[报文为空]]></return_msg></xml>";
/** * 该链接是通过【统一下单API】中提交的参数notify_url设置,如果链接无法访问,商户将无法接收到微信通知。 * 通知url必须为直接可访问的url,不能携带参数。示例:notify_url:“https://pay.weixin.qq.com/wxpay/pay.action” * <p> * 支付完成后,微信会把相关支付结果和用户信息发送给商户,商户需要接收处理,并返回应答。 * 对后台通知交互时,如果微信收到商户的应答不是成功或超时,微信认为通知失败,微信会通过一定的策略定期重新发起通知,尽可能提高通知的成功率,但微信不保证通知最终能成功。 * (通知频率为15/15/30/180/1800/1800/1800/1800/3600,单位:秒) * 注意:同样的通知可能会多次发送给商户系统。商户系统必须能够正确处理重复的通知。 * 推荐的做法是,当收到通知进行处理时,首先检查对应业务数据的状态,判断该通知是否已经处理过,如果没有处理过再进行处理,如果处理过直接返回结果成功。在对业务数据进行状态检查和处理之前,要采用数据锁进行并发控制,以避免函数重入造成的数据混乱。 * 特别提醒:商户系统对于支付结果通知的内容一定要做签名验证,防止数据泄漏导致出现“假通知”,造成资金损失。 */ @ApiOperation("支付回调") @RequestMapping("/pay/weixin/notify") public void wxNotify(HttpServletRequest request, HttpServletResponse response) { String resXml = ""; InputStream inStream; try { inStream = request.getInputStream(); ByteArrayOutputStream outSteam = new ByteArrayOutputStream(); byte[] buffer = new byte[1024]; int len; while ((len = inStream.read(buffer)) != -1) { outSteam.write(buffer, 0, len); } WXPayUtil.getLogger().info("wxnotify:微信支付----start----"); // 获取微信调用我们notify_url的返回信息 String result = new String(outSteam.toByteArray(), StandardCharsets.UTF_8); WXPayUtil.getLogger().info("wxnotify:微信支付----result----=" + result); // 关闭流 outSteam.close(); inStream.close(); // xml转换为map Map<String, String> map = WXPayUtil.xmlToMap(result); if (WXPayConstants.SUCCESS.equalsIgnoreCase(map.get("result_code"))) { WXPayUtil.getLogger().info("wxnotify:微信支付----返回成功"); if (WXPayUtil.isSignatureValid(map, wxProperties.getKey(), WXPayConstants.SignType.HMACSHA256)) { WXPayUtil.getLogger().info("wxnotify:微信支付----验证签名成功"); String orderNumber = map.get("out_trade_no"); // 订单支付完成,修改订单状态 //todo 当然是你自己的业务了,修改支付状态啊。 // 通知微信.异步确认成功.必写.不然会一直通知后台.八次之后就认为交易失败了. resXml = RES_SUCCESS_XML; } else { WXPayUtil.getLogger().warn("wxnotify:微信支付----判断签名错误"); resXml = RES_FAIL_XML; } } else { WXPayUtil.getLogger().warn("wxnotify:支付失败,错误信息:" + map.get("err_code_des")); resXml = RES_FAIL_XML; } } catch (Exception e) { WXPayUtil.getLogger().warn("wxnotify:支付回调发布异常:", e); } finally { try { // 处理业务完毕 BufferedOutputStream out = new BufferedOutputStream(response.getOutputStream()); out.write(resXml.getBytes()); out.flush(); out.close(); } catch (IOException e) { WXPayUtil.getLogger().error("wxnotify:支付回调发布异常:out:", e); } } }
|
好了,到这里就结束了,就是这么简单,你学会了吗?